Creating, and Commiting to, a new Gitlab Repository
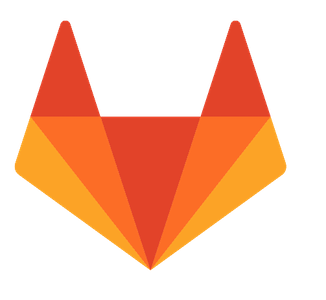
For more information on Gitlab, visit gitlab.com
Description
Gitlab is a fully featured GIT based repository server that allows users to among other things, to create and manage code repositories, track code issues, perform code reviews, define CICD pipelines, perform unit tests, create and manage docker registries, and much much more. This tutorial will walk through the steps required to create your first group, create your first project, and make your first commit on either your own Gitlab server, or to a repository on https://gitlab.com.
Pre-Requisites
1. Gitlab Server or Gitlab Account:
You must have an active Gitlab account. This account could be either be your own server, or can be an account on https://gitlab.com
Installing a Gitlab Server on AWS:
If you want to run your own gitlab server for the purpose of this lab, you can use the following CloudFormation template to launch a functional single server Gitlab instance. To get started, simply log into your AWS account and click the launch button below to start the stack deployment in the us-east-1 region:

2. GIT:
You must have GIT installed on your local workstation. For instructions on how to install GIT on your local workstation, go to the Git site, and follow the appropriate instructions for your computers platform. https://git-scm.com/downloads
Creating the Project
Before we are ready to commit some code to a repository, first we must create the location in Gitlab where we will keep the repository that we will ultimately be committing to, perform the following steps to create the project, and configure our repository to get ready for our first commit.
1. Create a Group:
The first thing we need to do is to set up a group, where we can place a new project, that ultimately will be the repository where we commit our code to. In order to do this, log into Gitlab, and click on the Create a group option from the main dashboard screen. If the button does not appear because you already have groups defined, then from the navigational menu, click on Groups, and then choose Explore groups. This will bring you to the Group view, where you can click on the New group button.
Once on the new group view, fill in the group details, and click on the Create group button.
2. Create the Project:
Once the group has been created, Click on the group, in this example being the Python group, and then click the New project button to create a new project, then fill in the project details, and click on the Create project button.
3. Copy Repo URL:
Once the project has been created, hit the Copy URL to clipboard icon to copy the git address to your clipboard.
Setup the Project Directory
Now that we have our repository created and configured, lets move over to our desktop to get some code ready for its first commit.
1. Create Project Directory:
The first thing that we need to do is to make a local directory on your local workstation where your git project can be constructed. Follow the steps for your platform to create a directory named SimpleAPI on our workstation and open a command shell, and navigate to the newly created directory.
Command:
mkdir -p ~/Documents/Projects/SimpleAPI cd ~/Documents/Projects/SimpleAPI
2. Initialize GIT:
Once in the new directory, we will want to initialize the directory to start tracking file changes that will be included in our git commits. In order to initialize a directory, simply type the following command.
Command:
git init
Command Console Output:
Initialized empty Git repository in /Users/awsdocs/Documents/Projects/SimpleAPI/.git/
3. Add the GIT Repo:
Now that we have GIT initialized, now we need to tell the local git repository what remote GIT repository to push to. We do this with the git remote add
command, combined with referencing the URL that we copied to our clipboard previously.
Command:
git remote add origin git@ec2-1-2-3-4.us-west-2.compute.amazonaws.com:Python/simpleAPI.git
NOTE:
The word origin between our command and the repo URL is the arbitrary name of the upstream remote repository. We name the remote repo locally, in the event that we want to add multiple upstream repositories to the local repository. For example, origin could be replaced with Gitlab, or Github, or any other identifiable remote git server/service identifier.
You can verify that the remote repository URL is set properly by looking at all remotely configured remote repositories for your local repository:
Command:
git remote -v
Command Console Output:
origin git@ec2-1-2-3-4.us-west-2.compute.amazonaws.com:Python/simpleAPI.git (fetch) origin git@ec2-1-2-3-4.us-west-2.compute.amazonaws.com:Python/simpleAPI.git (push)
Create the Code Base
1. Create API Python File:
Now that we have Git and our repositories all set up, we are ready to create a file named simpleAPI.py and paste the following code into the file.
# ******************************************************************* # Bottle Example API # Authors/Maintainers: Rich Nason (rnason@awsdocs.com) # ******************************************************************* # Required Modules: # ================= # Install Requrirements via PIP from bottle import Bottle, HTTPResponse # Webserver # Bottle Parameters: # =================== VERSION = '0.0.1' # API Version BOTTLEIP = '0.0.0.0' # The IP that Bottle will listen on to serve the API BOTTLEPORT = '80' # The TCP port that bottle will use to serve the API # Setup the Bottle WebServer Instance: # ==================================== APP = Bottle(__name__) # Index Route @APP.route('/', method=['OPTIONS', 'GET']) def index(): try: resp_msg = "All systems reporting go for launch!!" body = {'version': VERSION, 'message': resp_msg} response = HTTPResponse(status=200, body=body) return response except Exception as err: print(err) body = {'version': VERSION, 'message': err} response = HTTPResponse(status=400, body=body) return response # Start the Web Server # ===================== if __name__ == '__main__': try: SERVER = APP.run(host=BOTTLEIP, port=BOTTLEPORT, debug=True) except KeyboardInterrupt: pass print('exiting...') SERVER.stop()
Commit the Codes!
1. Commit:
Now that we have the directory, repositories and code file all set, its time to make our first commit. From the command line, simply use the git add --all
command to add all files in the current directory to the commit, and then use git commit -m "Message"
command to make our commit.
Command:
git add --all; git commit -m "Intial Code Commit"
Command Console Output:
[master (root-commit) d79b1f8] Intial Code Commit 1 files changed, 54 insertions(+) create mode 100644 simpleAPI.py
2. Push your Commit:
Last we need to simply push our commit(s) (Multiple commits can be pushed together). We do this simply by using git push
command.
GIT Command:
when using the git push
command, we need to supply 2 parameters.
- The name of the remote repository that we configured when we added the remote repository to the local repository.
- The branch name that we want to push to.
By default when we create a project we only have the master branch, however as the repository grows multiple branches can exist within the same repository.
Command:
git push origin master
Command Console Output:
Counting objects: 5, done. Delta compression using up to 4 threads. Compressing objects: 100% (5/5), done. Writing objects: 100% (5/5), 4.14 KiB | 0 bytes/s, done. Total 5 (delta 0), reused 0 (delta 0) To ec2-54-190-5-39.us-west-2.compute.amazonaws.com:Python/simpleAPI.git * [new branch] master -> master
3. Verify, and Do a Little Dance!:
Once the push has completed, you can log into Gitlab, click the project and you should see your new commit. Congratulations, you have successfully pushed your first commit to Gitlab!
Cleanup
Other than removing the local files from your workstation and deleting the repository, and group, there are no steps necessary in order to clean up or roll back the steps provided in this tutorial. In order to delete a project from Gitlab, simply navigate to the project/repository within the Gitlab console, Click on the the repository Settings, then under the General settings, click the Expand button in the Advanced section. Next, scroll to the bottom and select the very last option titled Remove project. This will envoke a dialog confirmation box. Type the name of the project/repository into the delete confirmation dialg and click Confirm. After this step, the repository and all history of it will be deleted.
Conclusion
This tutorial has gone over creating your first Gitlab group, project, and then finally the projects first commit. There are many additional things that we can do with Gitlab outside of the scope of this article. Future articles will cover Git flow, merge request stategy's, forking, and other topics that are relevant to the Git ecosystem. It is highly encouraged to poke around Gitlab futher and get used to the UI or CLI options to become a true Git Ninja. You can also add additional users to your Gitlab instance and grant those users various permissions on your repository to allow them to clone, branch and merge changes back to this mock codebase. "Git" coding and Enjoy !!
Gitlab Additional Resources
No Additional Resources.
Gitlab Site/Information References
No Additional References.