Containers 101 Lab
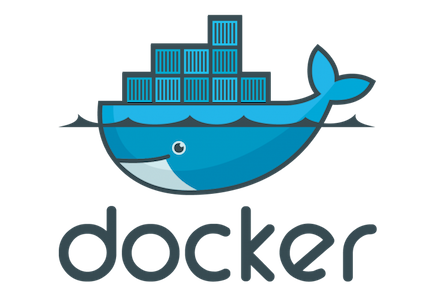
For more information on Docker, visit docker.com
Docker Description
This lab is meant to serve as a docker/containers 101 lab course. The objective of this lab will be to walk through a step by step exercise to help a user new to docker to get a docker image built, pushed to a registry, and deployed on a single non clustered standalone docker host.
Docker Pre-Requisites
Pre-Requisites Alternative:
As an alternative to installing the pre-requisites below, you could build an ec2-linux instance with all of the requirements bundled in. See the bottom of this page in the Resources section for a packer file that will create a lab builder AMI.
1. Docker for Mac or Docker for Windows:
The first thing that we will need to complete this lab will be to have a local docker environment running. This can be accomplished by either installing Docker for Mac, Docker for Windows, or the Docker engine installed on Linux.
2. AWS Account:
You will need to have an active AWS account, as this lab will cover pushing to an ECR (Elastic Container Registry), as well as deploying docker to an EC2 (Elastic Compute Cloud) instance.
3. IAM User:
You will need an IAM user created with a miniumum of EC2 and ECR permissions setup. The user should have programmatic access, and have a generated Access Key, and associated Access Secret Key.
4. Python and PIP:
You will need to have python and PIP (Pip Installs Packages) installed on your workstation so we can download and install the AWS CLI tools. This step will be required for obtaining ECR login credentials further down the tutorial.
- Python
yum/apt-get install -y python-pip
5. Install AWS CLI:
In order to interact with AWS easier, you should have the awscli tool installed and configured with proper user access and user access secrets. You can configure the access key and access secret key using the aws configure
command once the CLI tools have been installed via python pip
Command:
pip3 install awscli
aws configure AWS Access Key ID [None]: AWS Secret Access Key [None]: Default region name [None]: Default output format [None]:
Command Example:
Desktop$ aws configure AWS Access Key ID [None]: ABCDEFGHIJKLMNOPQRST AWS Secret Access Key [None]: **************************************** Default region name [us-east-2]: Default output format [None]:
6. Putty (Windows Only):
If your using windows, then you will need to have PuTTY and PuTTYGen installed on your local windows instance. We will need PuTTY in order to SSH to our docker instances, and PuTTYGen in order to convert the AWS Key PEM file to a PuTTY formatted PPK File.
- Download the AWS Key PEM file used to launch your instance to your local drive
- Follow the steps outlined in Managing Windows SSH Keys with PuTTY to convert the AWS PEM file to a PuTTY PPK formatted KeyPair.
7. Provision EC2 Instance and Install Docker:
Pre-Requisites Alternative:
The following step is required regardless of using your own workstation with the above pre-reqs installed, or using a separate EC2 bastion host for your build environment. The instance provisioned below will be used to deploy your built docker container image from the ECR registry
Instance Security Group
Provision an instance to run docker. The instance should have a security group that has SSH (port 22) and tcp port 8080 open.
Install Docker!
Docker Build Instance
You can build a docker instance by simply launching your favorite Linux distribution and using the built in package repository to install docker.
RHEL,
CentOS, &
Amazon Linux
Command:
sudo yum install docker
Now that we have Docker installed, we need to set Docker to start on boot.
Amazon Linux
sudo chkconfig docker on sudo /etc/init.d/docker start
RHEL & CentOS 7
systemctl enable docker.service
systemctl start docker.service
Debian, &
Ubuntu Linux
Command:
sudo apt-get update sudo sudo apt-get install apt-transport-https ca-certificates curl gnupg2 software-properties-common sudo curl -fsSL https://download.docker.com/linux/debian/gpg | sudo apt-key add - sudo add-apt-repository "deb [arch=amd64] https://download.docker.com/linux/debian $(lsb_release -cs) stable" sudo apt-get update sudo apt-get install docker-ce
Now that we have Docker installed, we need to set Docker to start on boot.
Debian & Ubuntu
sudo update-rc.d docker enable
8. Test Docker:
root@ip-172-31-7-255:~# docker ps --all CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
Creating a Docker Image
1. Create Dockerfile:
Open your favorite editor and create a file named Dockerfile. This file will contain a list of all instructions that are required to install your application and build a container image.
Copy the following Dockerfile content into your Dockerfile to build a Jenkins container image build. Note that if your using a remote EC2 instance, then you will need to use vim Dockerfile
and paste the content of the dockerfile below using ctl+p
, then close the file using esc
, :wq!
RHEL,
CentOS, &
Amazon Linux
############################################################ # Dockerfile to build Jenkins Base Container # Based on: centos:6 ############################################################ # Set the base image to Centos 6.6 Base FROM centos:6 # File Author / Maintainer MAINTAINER Rich Nason rnason@appcontainers.com ################################################################### #************************* APP VERSIONS ************************* ################################################################### ################################################################### #*************** OVERRIDE ENABLED ENV VARIABLES ***************** ################################################################### ################################################################### #****************** ADD REQUIRED APP FILES ********************** ################################################################### ################################################################### #******************* UPDATES & PRE-REQS ************************* ################################################################### # Run Update, and install Jenkins RUN yum clean all && \ yum -y update && \ yum -y install sudo java-1.8.0-openjdk java-1.8.0-openjdk-devel git wget openssh-server net-tools && \ export JAVA_HOME=/usr/lib/jvm/jre-1.8.0-openjdk.x86_64 && \ yum clean all && \ rm -fr /var/cache/* ################################################################### #******************* APPLICATION INSTALL ************************ ################################################################### # Add Jenkins Repository RUN cd /etc/yum.repos.d/ && \ wget -O /etc/yum.repos.d/jenkins.repo http://pkg.jenkins-ci.org/redhat/jenkins.repo && \ rpm --import https://jenkins-ci.org/redhat/jenkins-ci.org.key RUN yum clean all && \ yum -y install jenkins && \ yum clean all && \ rm -fr /var/cache/* ################################################################### #****************** POST DEPLOY CLEAN UP ************************ ################################################################### # Make SSH Directory, Instruct Jenkins not to prompt for host key verification and set perms RUN mkdir /var/lib/jenkins/.ssh && \ echo "Host *\n\tStrictHostKeyChecking no\n" >> /var/lib/jenkins/.ssh/config RUN mkdir -p /var/cache/jenkins/war || exit 0 && \ mkdir /var/log/jenkins || exit 0 && \ cd /var/cache/jenkins/war && \ jar -xvf /usr/lib/jenkins/jenkins.war && \ chmod a+w ./ # Reset Permissions RUN chown -R jenkins:jenkins /var/cache/jenkins && \ chown jenkins:jenkins /var/log/jenkins && \ chmod -R 775 /var/cache/jenkins && \ chmod -R 777 /var/log/jenkins && \ chown -R jenkins:jenkins /var/lib/jenkins && \ chown -R jenkins:jenkins /var/lib/jenkins/.ssh && \ chmod -R 0700 /var/lib/jenkins/.ssh && \ chmod -R 0600 /var/lib/jenkins/.ssh/* ################################################################### #***************** CONFIGURE START ITEMS ************************ ################################################################### RUN echo "service jenkins start" >> /root/.bashrc CMD /bin/bash ################################################################### #**************** EXPOSE APPLICATION PORTS ********************** ################################################################### # Expose ports to other containers only EXPOSE 8080 ################################################################### #******************* OPTIONAL / LEGACY ************************** ###################################################################
Debian, &
Ubuntu Linux
############################################################ # Dockerfile to build Jenkins Base Container # Based on: debian:stretch ############################################################ # Set the base image to Debian Jessie Base FROM debian:stretch # File Author / Maintainer MAINTAINER Rich Nason rnason@appcontainers.com ################################################################### #************************* APP VERSIONS ************************* ################################################################### ################################################################### #*************** OVERRIDE ENABLED ENV VARIABLES ***************** ################################################################### ################################################################### #****************** ADD REQUIRED APP FILES ********************** ################################################################### ################################################################### #******************* UPDATES & PRE-REQS ************************* ################################################################### # Run Update, and install Jenkins # Clean, Update, Upgrade, and Install... then clear non English local data. openjdk-7-jdk RUN apt-get clean && \ apt-get update && \ apt-get -y upgrade && \ apt-get -y install sudo git wget openssh-server net-tools gnupg2 openjdk-8-jdk && \ apt-get clean && \ rm -fr /var/lib/apt/lists/* ################################################################### #******************* APPLICATION INSTALL ************************ ################################################################### # Add Jenkins Repository RUN wget -q -O - http://pkg.jenkins-ci.org/debian/jenkins-ci.org.key | sudo apt-key add - && \ echo "deb http://pkg.jenkins-ci.org/debian binary/" >> /etc/apt/sources.list RUN apt-get clean && \ apt-get update && \ apt-get -y install jenkins && \ apt-get clean && \ rm -fr /var/lib/apt/lists/* ################################################################### #****************** POST DEPLOY CLEAN UP ************************ ################################################################### # Make SSH Directory, Instruct Jenkins not to prompt for host key verification and set perms RUN mkdir /var/lib/jenkins/.ssh && \ echo "Host *\n\tStrictHostKeyChecking no\n" >> /var/lib/jenkins/.ssh/config RUN mkdir -p /var/cache/jenkins/war || exit 0 && \ mkdir /var/log/jenkins || exit 0 && \ cd /var/cache/jenkins/war && \ jar -xvf /usr/lib/jenkins/jenkins.war && \ chmod a+w ./ # Reset Permissions RUN chown -R jenkins:jenkins /var/cache/jenkins && \ chown jenkins:jenkins /var/log/jenkins && \ chmod -R 775 /var/cache/jenkins && \ chmod -R 777 /var/log/jenkins && \ chown -R jenkins:jenkins /var/lib/jenkins && \ chown -R jenkins:jenkins /var/lib/jenkins/.ssh && \ chmod -R 0700 /var/lib/jenkins/.ssh && \ chmod -R 0600 /var/lib/jenkins/.ssh/* ################################################################### #***************** CONFIGURE START ITEMS ************************ ################################################################### RUN echo "service jenkins start" >> /root/.bashrc CMD /bin/bash ################################################################### #**************** EXPOSE APPLICATION PORTS ********************** ################################################################### # Expose ports to other containers only EXPOSE 8080 ################################################################### #******************* OPTIONAL / LEGACY ************************** ###################################################################
2. Build the Container:
Once the Dockerfile has been saved, you can use it to build a docker image that can be deployed to any environment. We will use the -t flag in the docker build statement to specify an initial tag for the image.
Command:
docker build -t lab/jenkins:latest .
NOTE:
- The docker build statement should be ran from the same directory where the Dockerfile was saved. The " . " at the end of the build statement is required, as it tells the Docker daemon to look in the current directory for a file named Dockerfile.
- Optionally you can use the -f flag to specify the location of the Dockerfile, in which case the Dockerfile does not need to be named Dockerfile as in the example of
docker build -t lab/jenkins:latest -f /tmp/myjenkinsfile
- The container will go through each step from the dockerfile being executed while the image is being built, and will display any console output generated from each step.
Command Console Output:
Removing intermediate container 98025c50a4d6 Step 8/11 : RUN chown -R jenkins:jenkins /var/cache/jenkins && chown jenkins:jenkins /var/log/jenkins && chmod -R 775 /var/cache/jenkins && chmod -R 777 /var/log/jenkins && chown -R jenkins:jenkins /var/lib/jenkins && chown -R jenkins:jenkins /var/lib/jenkins/.ssh && chmod -R 0700 /var/lib/jenkins/.ssh && chmod -R 0600 /var/lib/jenkins/.ssh/* ---> Running in 3fae508bab9a ---> 14ea938c3412 Removing intermediate container 3fae508bab9a Step 9/11 : RUN echo "service jenkins start" >> /root/.bashrc ---> Running in dfa20be95388 ---> 47181701e80c Removing intermediate container dfa20be95388 Step 10/11 : CMD /bin/bash ---> Running in 774ab02d3e59 ---> 37da3651ee62 Removing intermediate container 774ab02d3e59 Step 11/11 : EXPOSE 8080 ---> Running in 7214641391fd ---> c59152af0b38 Removing intermediate container 7214641391fd Successfully built c59152af0b38 Successfully tagged lab/jenkins:latest
3. Check the image:
When the image has been built successfully, Verify and Run the image. Once it's been ran, we can test its proper functionality by loading Jenkins in a browser.
Docker Images Command:
docker images
Command Console Output:
Desktop$ docker images REPOSITORY TAG IMAGE ID CREATED SIZE lab/jenkins latest c59152af0b38 10 minutes ago 603MB centos 6 7ea307891843 5 weeks ago 194MB
Docker Run Command:
docker run -it -d --name jenkins -h jenkins -p 8080:8080 lab/jenkins
Command Console Output:
Desktop$ docker ps --all CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 6810ca84f9ff lab/jenkins "/bin/sh -c /bin/bash" 8 seconds ago Up 7 seconds 0.0.0.0:8080->8080/tcp jenkins
Docker Run Flags:
In the run statement the following flags are used:
-it runs the container with an interactive tty.
--name will set the container name.
-h sets the hostname of the container.
-p is used to publish host ports and map them back to the corresponding container ports. (8080:8080 = host:container)
-d will launch the container in daemon mode. Without it, the container would launch, and put you directly into the shell. If you are put into the docker container shell, you can exit by pressing CTL P + CTL Q
.
4. Launch Jenkins:
Once the container has been launched open a web browser and go to the host bound port of 8080. The container build is successful if Jenkins loads properly in the browser.
5. Cleanup:
After Jenkins has been successfully launched in the browser we can now clean up the running container. We use the docker stop
command to stop the running instance, then use docker rm
to remove the running instance. Neither of these actions affect the image that we created, only the running instance of that image on our local machine. Once the running instance has been stopped and removed, we can push our tested image to the repository for future deployment.
Docker PS Command:
Desktop$ docker ps --all CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 9173a60282ed lab/jenkins "/bin/sh -c /bin/bash" 11 minutes ago Up 11 minutes 0.0.0.0:8080->8080/tcp jenkins
Docker Stop Command:
Desktop$ docker stop jenkins jenkins
Docker rm Command:
Desktop$ docker rm jenkins jenkins
Verify Container Command:
Desktop$ docker ps --all CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
Verify Images Command:
Desktop$ docker images REPOSITORY TAG IMAGE ID CREATED SIZE lab/jenkins latest c59152af0b38 10 minutes ago 603MB centos 6 7ea307891843 5 weeks ago 194MB
Pushing the Image to a Repository
Once the image has been built successfully, we will need a place to store the completed image so that we can deploy it out to our infrastructure. There are several available options for storing images into repositories, for this exercise we will be using Amazon ECR:
1. Log into your AWS Account, and go to the ECS console:
From the top left side of the navigational menu bar, click on the Services menu, and then choose Systems Manager by either navigating to the section of the listed services, or by typing the first few letters of the service name in the search box, and then choosing it from the filtered list.
2. Click Getting Started:
If you have never used ECS or ECR previously, you will be presented with the Getting Started page. Click the button. On the Getting Started screen, Uncheck the Deploy a sample application onto an Amazon ECS Cluster leaving only the Store container images securely with Amazon ECR option checked, and then press Continue.
3. Name the Registry:
Next we will need to name the registry. Type the name of your repository in the Repository Name field and then press Next Step.
Repository Name
Use only lower case letters and no special symbols in the container registry naming convention.
4. View Repo Instructions:
Last we will be presented with the information on how to log in and interact with our repository. Read through the instructions, and press the Done button once done.
Retrieving Login Instructions:
If you need to see the instructions again in the future, you can do so by going to the registry in the ECR Repositories section of the console, selecting the repository, and clicking the View Push Commands button at the top of the repository view window.
5. Get Login Creds:
On your workstation, we will now need to obtain the login command including the credentials for the ECR repository. In order to do so, perform the following command from your workstation. (You will need the AWS CLI tools for this step)
ECR Request:
aws ecr get-login --no-include-email --region us-east-2
Response:
docker login -u AWS -p eyJwYXlsb2FkIjoiZFlXTzlqUVRkdmxCcjZpaU0xSC9kT292YkR4RmhHMjRsZU9FNGs0czlGa1hzbmtmS05Cb3NqRjRmNTlZY1B4dkc3MU51bFU5eHBNZm8wczhGSUVHb2ljNnVwc0kzNVhkYzhBYjNHT2Z1RTVRMjByK09nLy9yOFlIYWdxWURkU1lLY3FlREh6YzBkazVyakxqRHViVXVXNUZGMjFVQUJ
sUkFqL3FHbGhSdmFKVXpkSStXdDQ2NHhIQmVQQWZCWmZzY2Y3UGRlMXVMV1dXK0Z2TEFSQnVySFA0UU8yQWl0RnpvSnFabFQ3Qnd6QTkwRW04MUxRWERWanplN0daOFN2SFR3cVNjVnloaXF2bEVpYUN1Z1RKU0hXQlpySWlReUJjU3diZkJaVEJPdWE0MS9pTXJqQXd1c3ZKOWFjVnpTUXFxZGZKZWZ0OXJLTE9NV21YRG9VdzI3R
HVhNWNKK1pUdW9WNnBIcVZLMld2QXIwR3Vod1F2UGU2SEx2L0U2YTBJOThmTmtkS1lZUE4wZXd1RmRnUWhQRy9RV0ZBek5DRml6bVJNa2lxZ0FBTnBacVdyTkVvUzJsWkh6K1JOaVBTOG5pSkxIMjlLV3VhcnhlanZwU2VoOEFJb3lIeGk2ZVRyb3FSaXc0Q1lDYkMyWThtQm1xT1dVb0RNTlhTM2dBY1NhNXlrSkhOaHdqRzl3WVd
nMkVQam5rY2FIcFJ6Y0VOWGZvZllPQ0laZmtmRHhIS0FHcDY4R21UT0doK0RFMWpSL2pQOGI2UmtuUUFpUXg2Q2JENEtsWUdCcUJQN0szeFl6Nm9ORVUrQ0xVTDRERjh0L3ZtSENReE5GOVlZWlMxQ3o5alFuTjc0VjR2V0d3N0hXOXJ5MjhZTHJkYkNyTFh5K0UvUkJJQ2VEYWNnMmw4MzBaK0JlMHpyNkxHbGE1SjFxTk0wbTVvO
DNjZ3hvZG10bTAvSjRoWWRpN25lQVVqZUt4UWdZVFBhWGliTHYxNG5Gbmg2bi8wZDYwWitXZGI2a203M3NJdkxrV1FnUng5bHJGd0NkTDhlRjd6REJ5MlBqaVlUNllZTEtHMVQrQnhMaUtLMUZxd2ZNRmoyVjBqOW1Tc3laQ29jYnhQTVozOGJidnV3OHdmRlVjWDdjaXZxNXpwaWU3S2Zoc2t6dnpDOHVTUjBJWisyV29RampJV1d
Idnc3MmxWU3NUQU05Y2xZRU0xaGxDUlZvbHE4ZGdaRkJVNytTdzcyQnVMcXI4Vks0STYwa3V6ZUxlbE5pVkIwdWtBaU9sUkZMNVRkWEFYMlA3ZkVNYThjSUgvUXp0eGIzcFhxZ1M5bGc0N1d2ZWpEVFlONXVBMTdIZVVpRFRseTZxT3pBV3d5K2Q5ZUFFNndWNkdmNmpCVEdmL0t0dFlYdThVUWdsY09pQ0djaVZoRWlocmhXejdie
GUvMDJKeHZiN0lCVjZCTmJVUzEiLCJkYXRha2V5IjoiQVFFQkFIajZsYzRYSUp3LzdsbjBIYzAwRE1lazZHRXhIQ2JZNFJJcFRNQ0k1OEluVXdBQUFINHdmQVlKS29aSWh2Y05BUWNHb0c4d2JRSUJBREJvQmdrcWhraUc5dzBCQndFd0hnWUpZSVpJQVdVREJBRXVNQkVFRExjK0FTYjhvNjNIZTJtR2N3SUJFSUE3RG8rM3h0NXd
TcXZMNy8zeUJhdEViRFlBQUIwN2hicm5kMG5lOXpjSWIwSXV6MUcwTFBtSEtsSTZ0NXdveE4rYlNHbklrOWVWUmFMSlFxdz0iLCJ2ZXJzaW9uIjoiMSIsInR5cGUiOiJEQVRBX0tFWSJ9
https://123456789012.dkr.ecr.us-west-2.amazonaws.com
ECR Region:
The above command references the us-west-2 region. Ensure that the region reflects the proper region where you created your repository.
6. Perform a docker login:
On your workstation, use the returned command response to log into your docker repository.
Syntax:
docker login -u AWS -p {{YOUR_LOGIN_KEY}}
Example Request:
docker login -u AWS -p eyJwYXlsb2FkIjoiZFlXTzlqUVRkdmxCcjZpaU0xSC9kT292YkR4RmhHMjRsZU9FNGs0czlGa1hzbmtmS05Cb3NqRjRmNTlZY1B4dkc3MU51bFU5eHBNZm8wczhGSUVHb2ljNnVwc0kzNVhkYzhBYjNHT2Z1RTVRMjByK09nLy9yOFlIYWdxWURkU1lLY3FlREh6YzBkazVyakxqRHViVXVXNUZGMjFVQUJ sUkFqL3FHbGhSdmFKVXpkSStXdDQ2NHhIQmVQQWZCWmZzY2Y3UGRlMXVMV1dXK0Z2TEFSQnVySFA0UU8yQWl0RnpvSnFabFQ3Qnd6QTkwRW04MUxRWERWanplN0daOFN2SFR3cVNjVnloaXF2bEVpYUN1Z1RKU0hXQlpySWlReUJjU3diZkJaVEJPdWE0MS9pTXJqQXd1c3ZKOWFjVnpTUXFxZGZKZWZ0OXJLTE9NV21YRG9VdzI3RHVhN WNKK1pUdW9WNnBIcVZLMld2QXIwR3Vod1F2UGU2SEx2L0U2YTBJOThmTmtkS1lZUE4wZXd1RmRnUWhQRy9RV0ZBek5DRml6bVJNa2lxZ0FBTnBacVdyTkVvUzJsWkh6K1JOaVBTOG5pSkxIMjlLV3VhcnhlanZwU2VoOEFJb3lIeGk2ZVRyb3FSaXc0Q1lDYkMyWThtQm1xT1dVb0RNTlhTM2dBY1NhNXlrSkhOaHdqRzl3WVdnMkVQam5 rY2FIcFJ6Y0VOWGZvZllPQ0laZmtmRHhIS0FHcDY4R21UT0doK0RFMWpSL2pQOGI2UmtuUUFpUXg2Q2JENEtsWUdCcUJQN0szeFl6Nm9ORVUrQ0xVTDRERjh0L3ZtSENReE5GOVlZWlMxQ3o5alFuTjc0VjR2V0d3N0hXOXJ5MjhZTHJkYkNyTFh5K0UvUkJJQ2VEYWNnMmw4MzBaK0JlMHpyNkxHbGE1SjFxTk0wbTVvODNjZ3hvZG10b TAvSjRoWWRpN25lQVVqZUt4UWdZVFBhWGliTHYxNG5Gbmg2bi8wZDYwWitXZGI2a203M3NJdkxrV1FnUng5bHJGd0NkTDhlRjd6REJ5MlBqaVlUNllZTEtHMVQrQnhMaUtLMUZxd2ZNRmoyVjBqOW1Tc3laQ29jYnhQTVozOGJidnV3OHdmRlVjWDdjaXZxNXpwaWU3S2Zoc2t6dnpDOHVTUjBJWisyV29RampJV1dIdnc3MmxWU3NUQU0 5Y2xZRU0xaGxDUlZvbHE4ZGdaRkJVNytTdzcyQnVMcXI4Vks0STYwa3V6ZUxlbE5pVkIwdWtBaU9sUkZMNVRkWEFYMlA3ZkVNYThjSUgvUXp0eGIzcFhxZ1M5bGc0N1d2ZWpEVFlONXVBMTdIZVVpRFRseTZxT3pBV3d5K2Q5ZUFFNndWNkdmNmpCVEdmL0t0dFlYdThVUWdsY09pQ0djaVZoRWlocmhXejdieGUvMDJKeHZiN0lCVjZCT mJVUzEiLCJkYXRha2V5IjoiQVFFQkFIajZsYzRYSUp3LzdsbjBIYzAwRE1lazZHRXhIQ2JZNFJJcFRNQ0k1OEluVXdBQUFINHdmQVlKS29aSWh2Y05BUWNHb0c4d2JRSUJBREJvQmdrcWhraUc5dzBCQndFd0hnWUpZSVpJQVdVREJBRXVNQkVFRExjK0FTYjhvNjNIZTJtR2N3SUJFSUE3RG8rM3h0NXdTcXZMNy8zeUJhdEViRFlBQUI wN2hicm5kMG5lOXpjSWIwSXV6MUcwTFBtSEtsSTZ0NXdveE4rYlNHbklrOWVWUmFMSlFxdz0iLCJ2ZXJzaW9uIjoiMSIsInR5cGUiOiJEQVRBX0tFWSJ9 https://123456789012.dkr.ecr.us-west-2.amazonaws.com
Example Response:
Login Succeeded
7. Tag the Image:
Tag the image with the repository name given in the ECR command list. This will allow us to push the image to the newly created ECR repository. An image tag consists of 3 components, the server location, repository name, and image build. In the case of this example the repository name of 123456789012.dkr.ecr.us-west-2.amazonaws.com/jenkins:latest breaks down telling the docker dameon to push the image to the server DNS name of 123456789012.dkr.ecr.us-west-2.amazonaws.com, using the jenkins repository, and flag it as the latest build.
Docker Image Tag:
Ensure when using the docker tag, and docker push statements that you replace the images tagged name, with the proper tag name from your own docker repository. The examples below reference the image and repository used during the creation of this lab, and are not valid tag or repository names.
Syntax:
docker tag lab/jenkins:latest {{REPO_ADDRESS}}/jenkins:latest
Example Request:
docker tag lab/jenkins:latest 123456789012.dkr.ecr.us-west-2.amazonaws.com/jenkins:latest
Once tagged run a docker images
command to verify that the image was tagged correctly:
docker images REPOSITORY TAG IMAGE ID CREATED SIZE 123456789012.dkr.ecr.us-west-2.amazonaws.com/jenkins latest a53da795f414 5 minutes ago 367MB lab/jenkins latest a53da795f414 5 minutes ago 367MB debian
8. Push the Image:
Now that we have both the Docker image and repository created, and are logged into the repository, we can now push the image to the repository.
Syntax:
docker push {{REPO_ADDRESS}}/jenkins
Example Request:
docker push 123456789012.dkr.ecr.us-west-2.amazonaws.com/jenkins
Example Response:
The push refers to a repository [123456789012.dkr.ecr.us-west-2.amazonaws.com/jenkins] 607f9d534485: Pushed 32eff9aeeadf: Pushed ed030e7c9ba6: Pushed 7b144cce2860: Pushed c77c4213307b: Pushed c8cf41c44d97: Pushed 5c86a415e035: Pushed 45f0f161f074: Pushed latest: digest: sha256:95de5123e73b78c41eb79e7b861b3726eb25c7735bb05a4488614f9380697202 size: 1989
9. Check the ECR Registry:
Once the image has been successfully pushed to the registry, we can verify it by going to the ECS/ECR console.
Deploying the Image to a Docker host
1. Log into your EC2 Docker Host:
This next section will cover deploying the docker image to a standalone Docker host, running on an Amazon EC2 Instance. SSH into your host, and ensure that Docker is running properly by issuing a docker info
command.
SSH Command:
ssh ec2-user@1.2.3.4
Command Console Output:
The authenticity of host '1.2.3.4 (1.2.3.4)' can't be established. ECDSA key fingerprint is SHA256:aaYjORsif3hmdbIHEBepYaQcvebOvEG+QJ3oHZzJZJo. Are you sure you want to continue connecting (yes/no)? yes Warning: Permanently added '1.2.3.4' (ECDSA) to the list of known hosts. __| __|_ ) _| ( / Amazon Linux AMI ___|\___|___| https://aws.amazon.com/amazon-linux-ami/2017.03-release-notes/ 8 package(s) needed for security, out of 8 available Run "sudo yum update" to apply all updates. [ec2-user@ip-172-31-1-61 ~]$ sudo su -
2. Test Docker:
Once successfully logged into docker, test the docker daemon to ensure its installed and running properly using the following few docker commands:
Docker Info Command:
docker info
Command Console Output:
Containers: 0 Running: 0 Paused: 0 Stopped: 0 Images: 0 Server Version: 17.03.2-ce Storage Driver: overlay2 Backing Filesystem: extfs Supports d_type: true Native Overlay Diff: true Logging Driver: json-file Cgroup Driver: cgroupfs Plugins: Volume: local Network: bridge host macvlan null overlay Swarm: inactive Runtimes: runc Default Runtime: runc Init Binary: docker-init containerd version: 4ab9917febca54791c5f071a9d1f404867857fcc runc version: 54296cf40ad8143b62dbcaa1d90e520a2136ddfe init version: 949e6fa Security Options: seccomp Profile: default Kernel Version: 4.9.38-16.35.amzn1.x86_64 Operating System: Amazon Linux AMI 2017.03 OSType: linux Architecture: x86_64 CPUs: 1 Total Memory: 993.5 MiB Name: ip-172-31-1-61 ID: M3IQ:2HUY:R375:MGSN:UW7C:A76W:74ZW:4DEG:L5L5:QPW7:IRYR:UJYK Docker Root Dir: /var/lib/docker Debug Mode (client): false Debug Mode (server): false Registry: https://index.docker.io/v1/ Experimental: false Insecure Registries: 127.0.0.0/8 Live Restore Enabled: false
Docker PS Command:
[root@ip-172-31-1-61 ~]# docker ps --all CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
3. Log into ECR:
Next we need to use the docker login
command previously obtained to log into the ECR registry from our new docker host. This will allow us access to the image that we built and pushed in the previous section of the lab.
Docker Image Tag:
Ensure when using the docker tag, and docker push statements that you replace the images tagged name, with the proper tag name from your own docker repository. The examples below reference the image and repository used during the creation of this lab, and are not valid tag or repository names.
Syntax:
docker login -u AWS -p {{YOUR_LOGIN_KEY}}
Example Request:
docker login -u AWS -p eyJwYXlsb2FkIjoiZFlXTzlqUVRkdmxCcjZpaU0xSC9kT292YkR4RmhHMjRsZU9FNGs0czlGa1hzbmtmS05Cb3NqRjRmNTlZY1B4dkc3MU51bFU5eHBNZm8wczhGSUVHb2ljNnVwc0kzNVhkYzhBYjNHT2Z1RTVRMjByK09nLy9yOFlIYWdxWURkU1lLY3FlREh6YzBkazVyakxqRHViVXVXNUZGMjFVQUJ sUkFqL3FHbGhSdmFKVXpkSStXdDQ2NHhIQmVQQWZCWmZzY2Y3UGRlMXVMV1dXK0Z2TEFSQnVySFA0UU8yQWl0RnpvSnFabFQ3Qnd6QTkwRW04MUxRWERWanplN0daOFN2SFR3cVNjVnloaXF2bEVpYUN1Z1RKU0hXQlpySWlReUJjU3diZkJaVEJPdWE0MS9pTXJqQXd1c3ZKOWFjVnpTUXFxZGZKZWZ0OXJLTE9NV21YRG9VdzI3RHVhN WNKK1pUdW9WNnBIcVZLMld2QXIwR3Vod1F2UGU2SEx2L0U2YTBJOThmTmtkS1lZUE4wZXd1RmRnUWhQRy9RV0ZBek5DRml6bVJNa2lxZ0FBTnBacVdyTkVvUzJsWkh6K1JOaVBTOG5pSkxIMjlLV3VhcnhlanZwU2VoOEFJb3lIeGk2ZVRyb3FSaXc0Q1lDYkMyWThtQm1xT1dVb0RNTlhTM2dBY1NhNXlrSkhOaHdqRzl3WVdnMkVQam5 rY2FIcFJ6Y0VOWGZvZllPQ0laZmtmRHhIS0FHcDY4R21UT0doK0RFMWpSL2pQOGI2UmtuUUFpUXg2Q2JENEtsWUdCcUJQN0szeFl6Nm9ORVUrQ0xVTDRERjh0L3ZtSENReE5GOVlZWlMxQ3o5alFuTjc0VjR2V0d3N0hXOXJ5MjhZTHJkYkNyTFh5K0UvUkJJQ2VEYWNnMmw4MzBaK0JlMHpyNkxHbGE1SjFxTk0wbTVvODNjZ3hvZG10b TAvSjRoWWRpN25lQVVqZUt4UWdZVFBhWGliTHYxNG5Gbmg2bi8wZDYwWitXZGI2a203M3NJdkxrV1FnUng5bHJGd0NkTDhlRjd6REJ5MlBqaVlUNllZTEtHMVQrQnhMaUtLMUZxd2ZNRmoyVjBqOW1Tc3laQ29jYnhQTVozOGJidnV3OHdmRlVjWDdjaXZxNXpwaWU3S2Zoc2t6dnpDOHVTUjBJWisyV29RampJV1dIdnc3MmxWU3NUQU0 5Y2xZRU0xaGxDUlZvbHE4ZGdaRkJVNytTdzcyQnVMcXI4Vks0STYwa3V6ZUxlbE5pVkIwdWtBaU9sUkZMNVRkWEFYMlA3ZkVNYThjSUgvUXp0eGIzcFhxZ1M5bGc0N1d2ZWpEVFlONXVBMTdIZVVpRFRseTZxT3pBV3d5K2Q5ZUFFNndWNkdmNmpCVEdmL0t0dFlYdThVUWdsY09pQ0djaVZoRWlocmhXejdieGUvMDJKeHZiN0lCVjZCT mJVUzEiLCJkYXRha2V5IjoiQVFFQkFIajZsYzRYSUp3LzdsbjBIYzAwRE1lazZHRXhIQ2JZNFJJcFRNQ0k1OEluVXdBQUFINHdmQVlKS29aSWh2Y05BUWNHb0c4d2JRSUJBREJvQmdrcWhraUc5dzBCQndFd0hnWUpZSVpJQVdVREJBRXVNQkVFRExjK0FTYjhvNjNIZTJtR2N3SUJFSUE3RG8rM3h0NXdTcXZMNy8zeUJhdEViRFlBQUI wN2hicm5kMG5lOXpjSWIwSXV6MUcwTFBtSEtsSTZ0NXdveE4rYlNHbklrOWVWUmFMSlFxdz0iLCJ2ZXJzaW9uIjoiMSIsInR5cGUiOiJEQVRBX0tFWSJ9 https://123456789012.dkr.ecr.us-west-2.amazonaws.com
Example Response:
Login Succeeded
4. Deploy the Image:
Once logged into ECR, we can now run the new image that we created earlier. There are 2 ways to get the image onto the new deployment host, The first would be to do a docker pull
, which would simply pull the image down to the host, requiring a second docker run
command to actually run it. With Docker however, the first step isn't required if we are running the image for the first time. Simply using the docker run
command will perform both steps automatically.
Syntax:
docker run -it -d --name jenkins -h jenkins -p 8080:8080 {{REPO_ADDRESS}}/jenkins:latest
Example Request:
docker run -it -d --name jenkins -h jenkins -p 8080:8080 123456789012.dkr.ecr.us-west-2.amazonaws.com/jenkins:latest
Example Response:
Unable to find image '123456789012.dkr.ecr.us-west-2.amazonaws.com/jenkins:latest' locally latest: Pulling from jenkins 40ae7ce86d93: Pull complete 7259c83d6d97: Pull complete 2c01368e2eff: Pull complete da849b4ff22f: Pull complete 2886258060bf: Pull complete a8c599f863b6: Pull complete affea2dd0686: Pull complete 8648a17db0a5: Pull complete Digest: sha256:95de5123e73b78c41eb79e7b861b3726eb25c7735bb05a4488614f9380697202 Status: Downloaded newer image for 123456789012.dkr.ecr.us-west-2.amazonaws.com/jenkins:latest 4d1a05e8341e764f2d4b47d04f47f16e07907846ae11d4315bc885be6258e720
Once the runs statement has completed, use the docker ps --all
command to verify that the container is running and that the status is up
Docker PS Command:
docker ps --all
Command Console Output:
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 4d1a05e8341e 123456789012.dkr.ecr.us-west-2.amazonaws.com/jenkins:latest "/bin/sh -c /bin/bash" 8 minutes ago Up 8 minutes 0.0.0.0:8080->8080/tcp jenkins
5. Test the Deployment:
Once the run has completed, we can test the deployment by opening a browser and going to the IP of the instance on port 8080. The Jenkins screen should appear.
Docker Additional Resources
Lab Packer Files:
Packer File:
{ "builders": [{ "type": "amazon-ebs", "access_key": "{{user `aws_access_key`}}", "secret_key": "{{user `aws_secret_key`}}", "region": "{{user `region`}}", "source_ami": "{{user `ami`}}", "instance_type": "{{user `instance_type`}}", "ami_name": "{{user `ami_name`}}-{{timestamp}}", "ami_description": "{{user `ami_description`}}", "availability_zone": "{{user `availability_zone`}}", "vpc_id": "{{user `vpc_id`}}", "subnet_id": "{{user `subnet_id`}}", "security_group_id": "{{user `security_group_id`}}", "ssh_keypair_name": "{{user `ssh_keypair_name`}}", "ssh_agent_auth": true, "ssh_username": "{{user `ssh_username`}}", "associate_public_ip_address": true, "ssh_private_ip": false, "tags": { "Name": "{{user `tag_name`}}", "OS_Version": "{{user `tag_osver`}}" } }], "provisioners": [ { "type": "shell", "inline": [ "sudo yum clean all", "sudo yum -y update", "sudo yum install -y docker jq", "sudo chkconfig docker on", "sudo /etc/init.d/docker start", "sudo pip install awscli", "sudo curl -LO https://storage.googleapis.com/kubernetes-release/release/$(curl -s https://storage.googleapis.com/kubernetes-release/release/stable.txt)/bin/linux/amd64/kubectl", "sudo mv kubectl /usr/bin/", "sudo chmod +x /usr/bin/kubectl", "sudo curl -L https://github.com/docker/compose/releases/download/1.16.1/docker-compose-`uname -s`-`uname -m` -o /usr/local/bin/docker-compose", "sudo chmod +x /usr/local/bin/docker-compose" ] } ]}
Packer Build-Vars File:
{ "aws_access_key": "ABCDEFGHIJKLMNOPQRST", "aws_secret_key": "abcdefghijklmnopqrstuvwxyz1234567890abcd", "instance_type": "t2.small", "region": "us-east-2", "availability_zone": "us-east-2a", "ami": "ami-ea87a78f", "vpc_id": "vpc-y12345ba", "subnet_id": "subnet-12a3456b", "security_group_id": "sg-a6ca00cd", "ssh_keypair_name": "MyKey", "ssh_username": "ec2-user", "ami_name": "Container-Lab", "ami_description": "Image with all of the tools required to run ECS/K8 Labs", "tag_name": "Container-Lab", "tag_osver": "Amazon Linux" }
Packer Build Command:
packer build -var-file=buildvars.json container_lab.json
Docker Site/Information References
No Additional References.